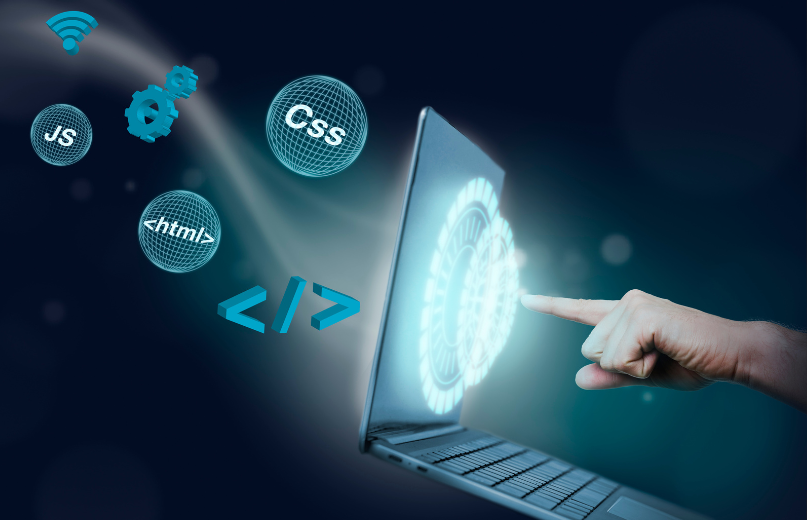
- November 11, 2022
- 333 Views
- 0 Likes
- IT Optimization
Optimizing Code: Best Practices for Faster and More Efficient Software
Introduction
In today’s fast-paced digital world, the performance of software applications is critical for delivering a seamless user experience. Slow and inefficient code can lead to frustrated users, increased hardware costs, and lost opportunities. Therefore, optimizing code for speed and efficiency is a fundamental aspect of software development. In this blog, we’ll explore some best practices and techniques to help developers write faster and more efficient code.
Use Efficient Data Structures and Algorithms
The choice of data structures and algorithms can significantly impact the performance of your software. Before implementing a solution, carefully analyze the problem requirements and choose the most appropriate data structures and algorithms for the task. For example, when dealing with large datasets, consider using hash tables for fast lookups, or employ sorting algorithms like quicksort or mergesort for efficient data manipulation.
Minimize Resource Consumption
Efficient code not only runs fast but also utilizes system resources judiciously. Avoid unnecessary memory allocation and deallocation, as excessive garbage collection can introduce significant overhead. Optimize your code to reuse objects whenever possible, and consider using object pools or caching mechanisms to reduce memory churn.
Limit Global Variables and Use Constants
Global variables can lead to unexpected side effects and make code maintenance challenging. Minimize their usage and favor local variables within functions. Additionally, use constants for fixed values that do not change during program execution, as they allow the compiler to perform optimizations and improve the code’s efficiency.
Profile Your Code
Profiling is a vital step in identifying performance bottlenecks. Use profiling tools to measure the execution time of various parts of your code and pinpoint areas that require optimization. By knowing which functions or sections are consuming the most resources, you can focus your efforts on the most critical areas to achieve maximum impact.
Employ Asynchronous and Parallel Processing
For tasks that involve waiting for I/O operations or performing computationally intensive tasks, consider using asynchronous programming or parallel processing. Asynchronous operations allow the CPU to handle other tasks while waiting for I/O to complete, improving overall efficiency. Parallel processing can leverage multiple cores and threads, providing significant speedup for CPU-bound tasks.
Optimize Loops and Conditionals
Loops and conditionals are core building blocks in programming, and optimizing them can have a substantial impact on performance. Minimize unnecessary iterations and condition checks within loops. Use the most efficient loop construct for the situation, such as a “for” loop for predictable iterations or a “while” loop for dynamic conditions.
Cache Data and Results
Caching can significantly speed up the execution of repetitive tasks or expensive computations. Identify parts of your code that generate the same results frequently and cache those results to avoid redundant processing. Utilize in-memory caching mechanisms or external caching systems to improve performance and reduce response times.
Optimize I/O Operations
I/O operations can often become a performance bottleneck, especially in applications dealing with large amounts of data. Use buffered I/O to minimize the number of disk reads and writes. Batch I/O operations when possible to reduce overhead, and avoid synchronous I/O calls that can block the execution flow.